ReferenceError: __dirname in ES Module Scope: Quick Fixes
To resolve the “ReferenceError: __dirname is not defined in ES module scope,” you must use `import.meta.url`. This error occurs because `__dirname` is a Node.js global available only in CommonJS modules, and you’re likely working within an ES module where it’s not defined.
Understanding the nuances of JavaScript, especially when dealing with module systems, can be tricky. The shift from CommonJS to ES Modules (ESM) has brought about significant changes in syntax and functionality. One such change is the way we reference the directory name of the current module.
Traditionally, Node. js developers have relied on the `__dirname` global variable to obtain this path in CommonJS modules. However, this convenience doesn’t directly translate to the ES Module system, leading to the aforementioned reference error. The solution involves adopting new ES Module features, specifically `import. meta. url`, to achieve similar functionality. This change underscores the evolving nature of JavaScript and highlights the importance of staying updated with the latest developments in the language. Adapting to these changes ensures your code remains robust and forward-compatible.
The Root Of Referenceerror: __dirname In Es Modules
Understanding the root of ReferenceError: __dirname in ES Modules is vital for modern Node.js developers. This error often confuses developers transitioning from CommonJS to ES Modules. Let’s explore what triggers this error and the key differences between ES Modules and CommonJS.
Common Scenarios Triggering The Error
- Using
__dirname
in ES Modules without a workaround - Importing a file with ES Module syntax in a CommonJS module
- Misconfiguring a build tool or bundler that does not shim
__dirname
Es Modules Vs. Commonjs: Key Differences
Feature | ES Modules | CommonJS |
---|---|---|
Syntax | import/export |
require/module.exports |
Loading | Asynchronous | Synchronous |
Variables | No __dirname or __filename |
Includes __dirname and __filename |
In ES Modules, __dirname
is not defined by default. This is unlike CommonJS, where it is a global variable. Developers must use modern alternatives or workarounds in ES Modules.

Credit: iamwebwiz.medium.com
Quick Fixes For Referenceerror: __dirname
Encountering a ReferenceError for __dirname in ES modules can be frustrating. Developers transitioning from CommonJS often face this issue. In ES modules, __dirname is not defined. This leads to errors when running code that relies on it. But there’s no need to worry. We’ll explore quick fixes to get your code running smoothly again. Each method is simple and effective.
Using
import.meta.urlThe import.meta.url property provides the current module’s URL. It’s a handy way to get the directory name. Let’s see how to use it:
- First, set the module’s URL to a variable.
- Then, use new URL() to process it.
- Finally, extract the directory path.
const directory = new URL('.', import.meta.url).pathname;
Path Module And Url Constructor Combo
Combine Node.js path module with the URL constructor. This creates a robust solution. Follow these steps:
- Import the path module using import statement.
- Create a URL instance from import.meta.url.
- Use path.dirname() to get the directory name.
import path from 'path';
const __dirname = path.dirname(new URL(import.meta.url).pathname);
These methods ensure your code runs without __dirname errors. They also maintain a clean and modern codebase.
Implementing Import.meta.url For Directory Paths
Implementing import.meta.url is essential in modern JavaScript. Developers often use ES modules in Node.js. Here, the traditional __dirname
is undefined. Instead, import.meta.url
provides the current module’s path.
Code Snippet: Resolving __dirname
Let’s replace __dirname
with import.meta.url
. This approach works well in ES modules. Below is a code snippet to demonstrate this:
import { fileURLToPath } from 'url';
import { dirname } from 'path';
const __filename = fileURLToPath(import.meta.url);
const __dirname = dirname(__filename);
console.log(__dirname); // Outputs the directory path
Potential Pitfalls And How To Avoid Them
Developers may face issues while implementing import.meta.url
. Below are common pitfalls:
- Path format – Ensure the URL is converted to a file path.
- Compatibility – Use only in ES modules, not CommonJS.
- Relative paths – Resolve paths relative to the module.
Here are steps to avoid these issues:
- Use
fileURLToPath
to convert URLs to paths. - Check the module system in use before implementation.
- Use
dirname
from the ‘path’ module to ensure correct paths.
Alternative Solutions And Workarounds
Encountering the ‘ReferenceError: __dirname is not defined in ES module scope’ can be puzzling. Developers often face this error when working with ES modules in Node.js. The solutions lie in understanding the module system and finding the right workaround.
Utilizing Third-party Modules
One way to bypass the ‘__dirname’ issue is using third-party modules. These modules simulate the CommonJS variables.
import-meta-resolve
– Helps in resolving paths.path
module – Offers directory name resolution.
Integrate these modules into your code for smooth functionality.
Example:
import path from 'path';
const __dirname = path.resolve();
console.log(__dirname); // Outputs the directory name
Reverting To Commonjs For Specific Files
Sometimes, the simplest solution is to revert to CommonJS. This is for files that need ‘__dirname’.
- Rename your file from
.js
to.cjs
. - Use CommonJS syntax in these files.
This change allows you to use ‘__dirname’ without errors.
Example:
// In a .cjs file
console.log(__dirname); // Outputs the directory name
With these workarounds, you can avoid the ‘__dirname’ error and maintain your workflow efficiently.
Best Practices When Dealing With Es Modules
Best Practices When Dealing with ES Modules can enhance your JavaScript projects. They let you manage and encapsulate code efficiently. However, they work differently than traditional CommonJS modules. This can lead to errors like ReferenceError: __dirname is not defined in ES module scope
. Let’s explore how to avoid such issues and make the most of ES Modules.
Consistent Module Syntax
Choose either ES Modules or CommonJS. Mixing both can cause confusion and errors. Stick to one format for your entire project. This creates a stable and predictable environment for your code to run.
- Use
import
andexport
for ES Modules. - Use
require
andmodule.exports
for CommonJS.
Understanding Es Module Limitations
ES Modules do not have built-in objects like __dirname
and __filename
. These are part of CommonJS. Knowing this prevents errors and promotes better code practices.
- Do not use
__dirname
in ES Modules. - Use new ES Module features like
import.meta.url
to get directory info.
Remember, ES Modules are static. They are easier to analyze and optimize by tools. This leads to better performance and easier debugging.
Adopt these best practices to avoid common pitfalls. Your code will be more efficient and less error-prone.
Common Errors To Avoid
When working with Node.js and ES modules, certain pitfalls can lead to the ReferenceError: __dirname is not defined. It’s crucial to avoid these common mistakes.
Mixing Module Types
Using both CommonJS and ES modules together can cause issues. Stick to one to prevent errors. Here’s what to look out for:
- Consistency: Choose either CommonJS or ES modules for your project.
- Imports: Use
import
for ES modules,require()
for CommonJS. - Exports: Match
export
statements with module types.
Check your code to ensure correct usage of module syntax.
Ignoring Async Import Implications
ES modules are asynchronous. This affects how modules load. Keep in mind:
- Top-level Code: Wrap it in async functions if it relies on imports.
- Dynamic Imports: Use
await
or.then()
to handle them properly.
Understanding async behavior is key to using ES modules effectively.
Tools And Libraries To Simplify Your Workflow
Dealing with ReferenceError: __dirname is not defined in ES module scope? This error can halt your project. But, fear not! Certain tools and libraries can streamline your workflow. They make handling paths and converting modules a breeze.
Recommended Libraries For Path Handling
Path handling is vital in any Node.js project. Libraries come to the rescue. They ensure smooth file and directory manipulations.
- path – Core Node.js module for file paths
- path-browserify – Browser version for path operations
These libraries provide functions like join()
and resolve()
. They replace __dirname in modules.
Development Tools For Module Conversion
Converting scripts to ES modules is simpler with the right tools. They help fix ReferenceError: __dirname.
- Webpack – Bundles and converts scripts
- Babel – Transforms syntax for compatibility
- esm – Enables ES module loader in Node.js
Using these, you can transform commonJS modules. This allows use of import/export
syntax without errors.
Credit: github.com
Future-proofing Your Javascript Projects
Future-proofing your JavaScript projects means preparing for changes. JavaScript evolves, and so do the standards. Developers encounter errors like ReferenceError: __dirname is not defined in ES module scope
. This suggests a need to adapt to the latest modules and practices.
Staying Updated With Ecmascript Standards
ECMAScript standards guide the evolution of JavaScript. Staying updated ensures your code stays robust. Follow new releases and features. Use compatibility tables to check browser support. Adopt best practices to keep your codebase healthy.
The Role Of Build Tools And Transpilers
Build tools and transpilers are essential in a modern developer’s toolbox. They transform new syntax to compatible code. Tools like Webpack and Babel help manage project dependencies and support older environments.
- Use build tools to bundle modules.
- Transpile code for broader compatibility.
- Stay ahead with continuous integration.
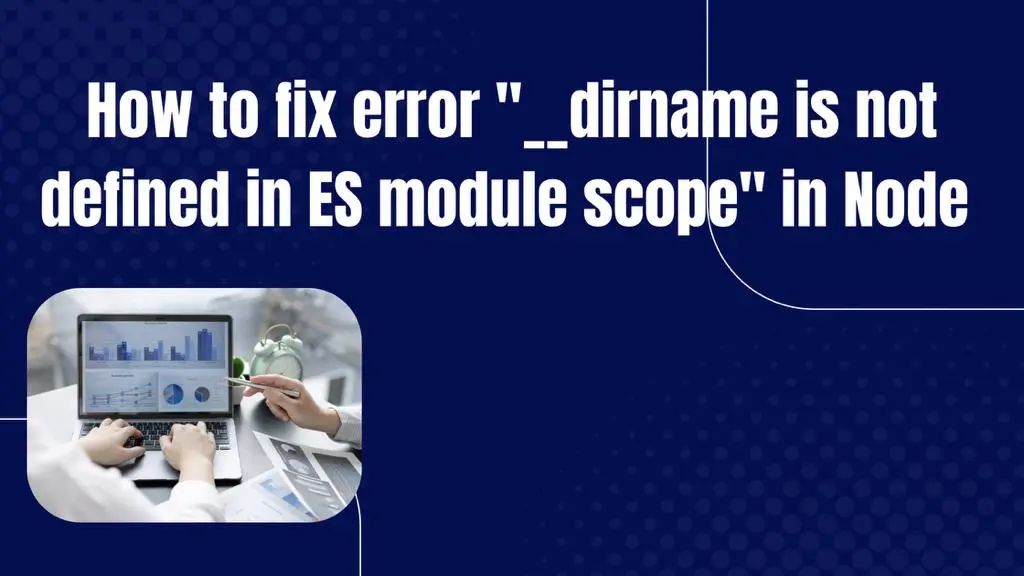
Credit: codebeautify.org
Frequently Asked Questions
How To Fix __ Dirname Is Not Defined?
To fix “__dirname is not defined,” ensure you’re using a Node. js environment, as “__dirname” is specific to Node. For modules, use “import. meta. url” to achieve similar functionality. Always check your code’s context and target environment compatibility.
How To Get __ Dirname In Es Module?
To obtain `__dirname` in an ES module, use `import. meta. url` and `new URL` like so: `const __dirname = new URL(‘. ‘, import. meta. url). pathname;`.
How To Define Dirname?
To define dirname, it’s the path to a file’s parent directory. This term is commonly used in programming to locate files efficiently. Understanding dirname helps in managing file systems and navigating directories effectively.
What Is The Es Module In Javascript?
The ES module, short for ECMAScript Module, is JavaScript’s official standard for importing and exporting code. This system allows developers to organize code into reusable modules. ES modules enhance code maintainability and project scalability.
Conclusion
Navigating the intricacies of ES Modules can be challenging, especially with __dirname errors. Remember, solutions exist to tackle this issue effectively. Embrace the modern syntax, utilize available tools, and consult documentation to enhance your coding journey. Stay informed, code smartly, and watch your projects thrive.